5 users have rated this article. result: |
|
4.4 out of 5. |
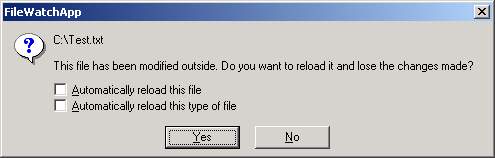
Introduction
This class helps you to monitor files like in the DevStudio. If the file is
modified outside your application a message box pops up and lets you choose between ignoring modifications or reloading the data,
thus discarding all changes.
If the file is modified a message is sent either to the specified view or to the first view of a specified document. The
message handler will call the reload function of the document class. The class should be thread
safe.
Things you have to change in your View Class and Document Class:
In your document class header file:
class CFileWatchAppDoc : public CRichEditDoc
{
....
public:
void OnFileReload();
protected:
DWORD m_hFileWatch;
void AddToFileWatch();
};
In your document class source file:
#include "FileWatch.h"
CFileWatchAppDoc::CFileWatchAppDoc()
{
m_hFileWatch = NULL;
}
CFileWatchAppDoc::~CFileWatchAppDoc()
{
CFileWatch::RemoveHandle(m_hFileWatch);
}
BOOL CFileWatchAppDoc::OnSaveDocument(LPCTSTR lpszPathName)
{
CFileWatch::RemoveHandle(m_hFileWatch);
BOOL bSuccess = CRichEditDoc::OnSaveDocument(lpszPathName);
AddToFileWatch();
return bSuccess;
}
void CFileWatchAppDoc::SetPathName(LPCTSTR lpszPathName, BOOL bAddToMRU)
{
CFileWatch::RemoveHandle(m_hFileWatch);
CRichEditDoc::SetPathName(lpszPathName, bAddToMRU);
AddToFileWatch();
}
void CFileWatchAppDoc::AddToFileWatch()
{
m_hFileWatch = CFileWatch::AddFileFolder(lpszPathName,
NULL, this);
CFileWatch::SetAutoReload(m_hFileWatch,
&((CYourApp*)AfxGetApp())->m_bAutoReloadDocTypeXY);
}
void CFileWatchAppDoc::OnFileReload()
{
...
UpdateAllViews(NULL);
}
In your view class header file:
class CFileWatchAppView : public CRichEditView
{
...
protected:
afx_msg LRESULT OnFileWatchNotification(WPARAM wParam,
LPARAM lParam);
DECLARE_MESSAGE_MAP()
};
In your view class source file:
#include "FileWatch.h"
BEGIN_MESSAGE_MAP(CFileWatchAppView, CRichEditView)
ON_REGISTERED_MESSAGE(CFileWatch::m_msgFileWatchNotify,
OnFileWatchNotification)
END_MESSAGE_MAP()
LRESULT CFileWatchAppView::OnFileWatchNotification(WPARAM wParam,
LPARAM lParam)
{
CString sPathName = CString((LPCTSTR)lParam);
DWORD dwData = (DWORD)wParam;
GetDocument()->OnFileReload();
return 0;
}
In your mainframe class source file:
#include "FileWatch.h"
void CMainFrame::OnClose()
{
CFileWatch::Stop();
CMDIFrameWnd::OnClose();
}
Add Non-document files to the watch:
Non-document files, which should be monitored, can be added to the watch but must be associated with a view class. Use
DWORD dwData
or the file name to distinguish which file has been changed.
#include "FileWatch.h"
void class::xy()
{
...
DWORD hHandle = CFileWatch::AddFileFolder(LPCTSTR lpszFileName,
HWND hWnd, CDocument* pDocument=NULL, DWORD dwData);
...
}
LRESULT CYourView::OnFileWatchNotification(WPARAM wParam,
LPARAM lParam)
{
CString sPathName = CString((LPCTSTR)lParam);
DWORD dwData = (DWORD)wParam;
if (sPathName == GetDocument()->GetPathName())
GetDocument()->OnFileReload();
else
...
return 0;
}
Note:
Not all file systems record write time in the same manner. For example, on Windows NT FAT, create time has a resolution of 10 milliseconds, write time has a resolution of 2 seconds, and access time has a resolution of 1 day (really, the access date). On NTFS, access time has a resolution of 1 hour. Furthermore, FAT records times on disk in local time, while NTFS records times on disk in UTC, so it is not affected by changes in time zone or daylight saving time. I have no information about the resolution of the write time in FAT or FAT32. However, if the file is modified several times in the same time window, only one notification can be detected!
Download latest version here.
Hint: For improved responsiveness, use Internet Explorer 4 (or above) with Javascript enabled, choose 'Use DHTML' from the View dropdown and hit 'Set Options'. | New thread | Messages 1 to 1 of 1 (Total: 1) | First Prev Next Last |
| |  | Author |  |
|  | Date |  |
|
 |  | | qweqwe | 14:01 15 Jun 01 |
 |
| Last Visit: 12:00 Friday 1st January, 1999 | First Prev Next Last |
|
|